Welcome! Today I am gonna go over some of the basics of starting with Android app development.
Chapter 1: Default methods
In Android you have several default methods you can use to ease the use.
The main method is onCreate.
this method goes like so:
This method is called when and activity is launched. An activity can be launched either on the start of the application or by typing this code
Another default method you will see when first creating a application is the onCreateOptionsMenu.
this method goes like so:
Along with this method comes the on onOptionsItemSelected method.
this method goes like so:
Chapter 2: Getting input from the user and building our first GUI.
In Android Studio the designer is very easy to grasp. It is really just drag and drop.
One tip you should ALWAYS follow is decent naming conventions. Look here at Google's guidelines.
So lets add a Button and an EditText. Lets name the Button "btnTest" and EditText "edtTxt".
Your XML code will look like so:
Now lets setup a onClickListener so that we can do something after we click the button!
the methods we are gonna use onSetClickListener, onClick and onClickListener and findViewById
code goes like so:
I will continue this later
Chapter 1: Default methods
In Android you have several default methods you can use to ease the use.
The main method is onCreate.
this method goes like so:
PHP:
@Override
protected void onCreate(Bundle savedInstanceState){
super.OnCreate(savedInstanceState); //If there is a saved state it will continue
setContentView(R.layout.activity_main); //Set the layout.
}
This method is called when and activity is launched. An activity can be launched either on the start of the application or by typing this code
PHP:
Intent i = new Intent(ThisClass.this, NextClass.class);
ThisClass.this.startActivity(i);
Another default method you will see when first creating a application is the onCreateOptionsMenu.
this method goes like so:
PHP:
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu_main, menu); //The name of this menu may change depending on your naming, but this inflates a menu which is shown when using the menu button -_-.
return true; //return true to popup.
}
Along with this method comes the on onOptionsItemSelected method.
this method goes like so:
PHP:
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId(); //Get the ID of the selected item and setup the if or if you wanna get cleaner switch(case).
if (id == R.id.action_settings) {
//If you have a setting page you can do the following from above to start it.
Intent i = new Intent(ThisClass.this, Settings.class);
ThisClass.this.startActivity(i);
return true;
}
return super.onOptionsItemSelected(item);
}
Chapter 2: Getting input from the user and building our first GUI.
In Android Studio the designer is very easy to grasp. It is really just drag and drop.
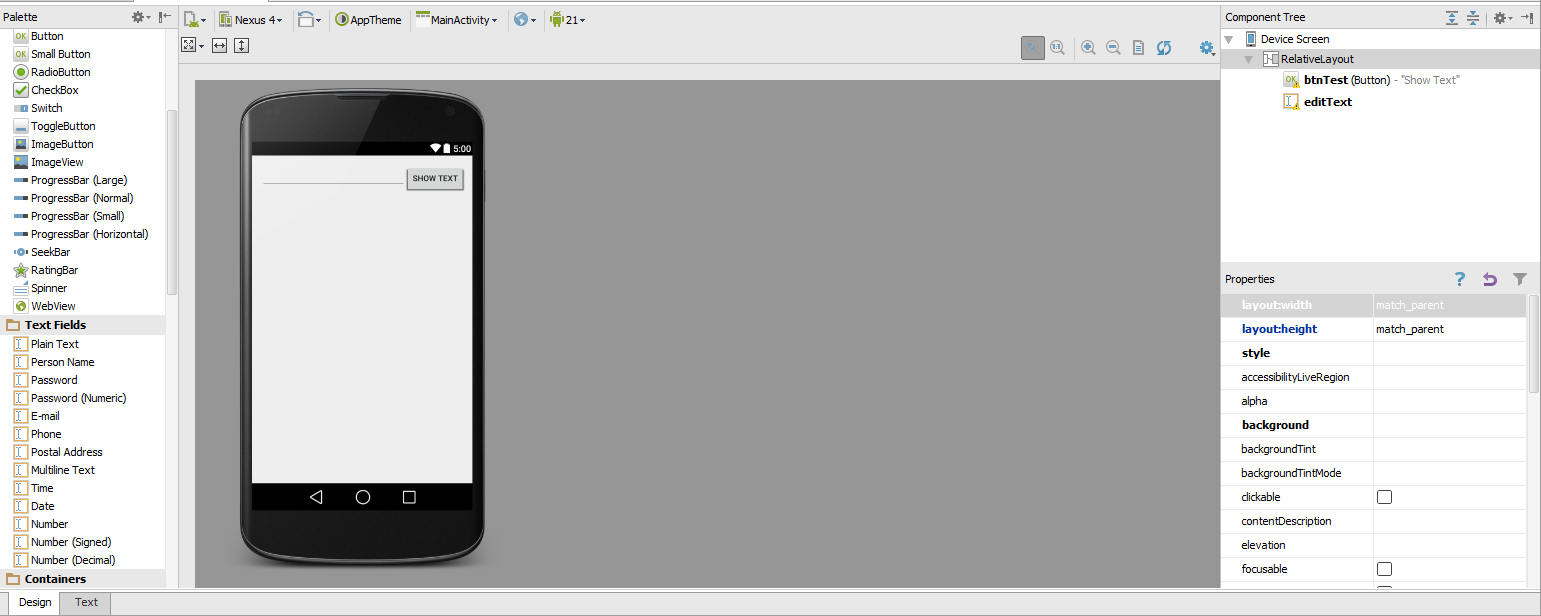
One tip you should ALWAYS follow is decent naming conventions. Look here at Google's guidelines.
So lets add a Button and an EditText. Lets name the Button "btnTest" and EditText "edtTxt".
Your XML code will look like so:
PHP:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Show Text"
android:id="@+id/btnTest"
android:layout_alignParentTop="true"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true" />
<EditText
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/edtTxt"
android:layout_alignParentTop="true"
android:layout_toLeftOf="@+id/btnTest"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true" />
</RelativeLayout>
Now lets setup a onClickListener so that we can do something after we click the button!
the methods we are gonna use onSetClickListener, onClick and onClickListener and findViewById
code goes like so:
PHP:
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button btn = (Button)findViewById(R.id.btnTest); //Cast the widget and find it.
EditText et = (EditText)findViewById(R.id.edtTxt); //Cast the widget and find it.
btn.setOnClickListener( //Set the buttons onClickListener
new View.OnClickListener() {
@Override
public void onClick(View v) { //onClick method
//Run code here.
Toast.makeText(MainActivity.this, "Text in the EditText: " + et.getText(), Toast.LENGTH_LONG).show(); //This will make a hint at the bottom.
}
}
);
}
I will continue this later